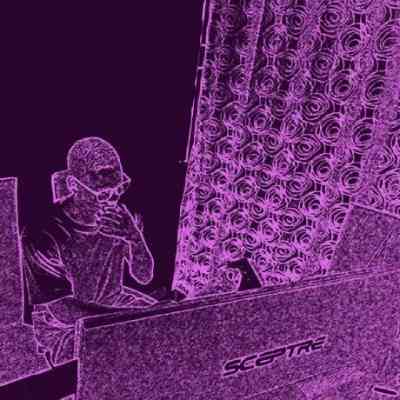
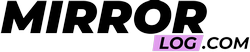
{{ post.title }} Laravel Tips And Guides
More in "Laravel Tips And Guides" Series
- Laravel Tutorial: Setup Supervisor Queue in 2025
- Never Hit Timeout Again: Using Laravel Queue Jobs for Large Data Exports
- You're tackling cross-platform data migration. How can you safeguard against security vulnerabilitie ...Read more
- Laravel Migration Best Practices: Mastering Database Schema Management
- Why do I need to run php artisan optimize every time I add a new route or update my code?
- Laravel Application Security: Tokens vs. API Keys - What's the difference?
-
Shoutout to Laravel for raising a $57M Series A in partnership with Accel. Laravel Cloud will soon b ...Read more
-
Stop Obsessing Over Clean Code: Here’s How to Deliver Fast and Stay Clean Enough
-
Enhance Laravel Development with Telescope: A Must-Have Tool
- Laravel Query Optimization: Choosing Between select() and get()
- Forget Best Practices? How to Find & Fix Your Laravel App's Slow Spots
- How to Optimize Your Web Application
-
Laravel Eloquent: Stop Killing Your Database (and Your Patience)
-
Laravel Ultimate Guide - What You Need To Know
Be the first to comment...
{{ commentCount }} {{ commentCount == 1 ? 'comment' : 'comments' }}