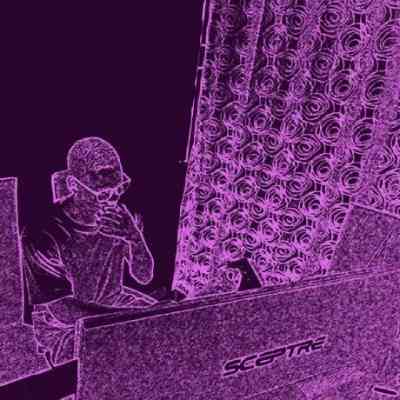
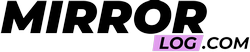
Laravel Migration Best Practices: Mastering Database Schema Management
Understanding Laravel Migrations: A Comprehensive Guide
Are you a Laravel developer struggling with database migrations? You're not alone. Proper management of Laravel migrations is crucial for maintaining a robust and scalable application. In this comprehensive guide, we'll dive deep into Laravel migration best practices, common pitfalls, and expert solutions to level up your development skills.
The Importance of Laravel Migrations in Web Development
Laravel migrations are a powerful feature that allows developers to version control their database schema. They act as a blueprint for your database structure, enabling seamless collaboration and consistent deployments across different environments. However, mishandling migrations can lead to severe consequences for your project.
Common Laravel Migration Mistakes: What to Avoid
The Temptation: Editing Existing Migration Files
Picture this scenario:
Junior Dev: "I added a column in the existing table migrations file and ran 'php artisan migrate:fresh'. Productive day, right?"
Team Lead: "Did you just go back in time to change your birth certificate?"
This approach, while tempting, is a recipe for disaster. Let's break down why:
- Loss of Database History: Each migration represents a step in your schema's evolution. Altering old migrations erases this valuable history.
- Team Collaboration Nightmares: In a multi-developer environment, this practice can lead to inconsistent database states across the team.
- Deployment Disasters: Your production database may end up in an unexpected state during deployments.
Laravel Migration Best Practices: The Right Way to Manage Your Database Schema
- Create New Migration Files for Every Change
Always use the Laravel Artisan command to generate a new migration:
```
php artisan make:migration add_column_to_table_name
``` - Implement Both 'Up' and 'Down' Methods
Ensure your migration is reversible by properly implementing both methods:
```
public function up()
{
Schema::table('users', function (Blueprint $table) {
$table->string('new_column');
});
}
public function down()
{
Schema::table('users', function (Blueprint $table) {
$table->dropColumn('new_column');
});
}
``` - Use 'php artisan migrate' Instead of 'migrate:fresh'
Apply new migrations without resetting your entire database:
```
php artisan migrate
``` - Leverage Laravel's Schema Builder
Utilize Laravel's powerful Schema Builder for creating and modifying tables:
```
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
```
Advanced Laravel Migration Techniques
- Database Seeding for Testing and Development
Use Laravel's seeding feature to populate your database with test data:
```
php artisan make:seeder UsersTableSeeder
``` - Migration Rollbacks and Resets
Learn to use rollbacks for undoing migrations:
```
php artisan migrate:rollback
``` - Database Transactions in Migrations
Wrap your migrations in transactions for added safety:
```
DB::transaction(function () {
// Your migration logic here
});
```
Mastering Laravel migrations is a crucial step in becoming a proficient Laravel developer. By following these best practices, you'll ensure your database schema remains manageable, your team collaboration smooth, and your deployments worry-free.
Remember, great Laravel development isn't just about writing code that works today—it's about setting up your project for long-term success and scalability.
Share Your Laravel Migration Experiences
Have you encountered challenging migration scenarios? Share your experiences or tips in the comments below. Let's learn from each other and elevate the Laravel community together!
0
comments
Follow creator