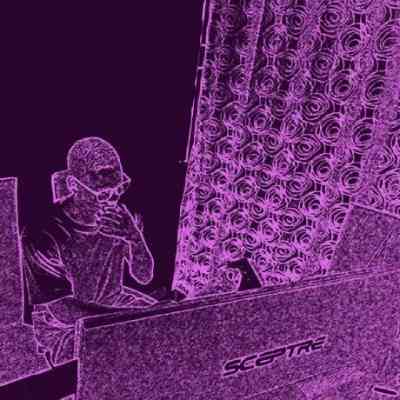
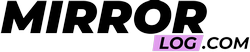
Laravel Query Optimization: Choosing Between select() and get()
Hey Laravel Developers,
Did you know you can pass column names directly to get() in Laravel?
However, it might not always be the best choice for your queries.
Why get() method might not be the perfect choice:
- The select() method is more flexible. You can chain other query methods easily.
- It's clearer to read, especially for complex queries.
- Using select() allows for better query optimization in some cases.
When to choose between select() and get() methods:
- Use get(['columns']) for quick, simple queries.
- Stick with select() for complex queries or when you need to chain methods.
Example code showing the difference between select() and get() method:
```
1. Flexibility
// Using select()
$recentPosts = Post::select('id', 'title', 'content')
->where('status', 'published')
->orderBy('created_at', 'desc')
->take(5)
->get();
// Using get()
$posts = Post::get(['id', 'title', 'content']);
// Filtering and sorting must be done after fetching all data
$recentPosts = $posts->where('status', 'published')
->sortByDesc('created_at')
->take(5)
->values();
2. Readability for complex queries
// Using select() with a join
$popularPosts = Post::select('posts.id', 'posts.title', 'users.name as author')
->join('users', 'posts.user_id', '=', 'users.id')
->where('posts.views', '>', 1000)
->orderBy('posts.views', 'desc')
->take(10)
->get();
// Using get() with a join (less readable and efficient)
$allPosts = Post::with('user')->get(['id', 'title', 'user_id']);
$popularPosts = $allPosts->filter(function($post) {
return $post->views > 1000;
})->sortByDesc('views')->take(10)->map(function($post) {
return [
'id' => $post->id,
'title' => $post->title,
'author' => $post->user->name
];
});
3. Query optimization
// Using select() to fetch only necessary columns
$optimizedQuery = Post::select('id', 'title')
->where('status', 'published')
->whereDate('created_at', '>', now()->subDays(30))
->orderBy('created_at', 'desc')
->limit(20)
->get();
// Using get() fetches all columns, which might be less efficient
$lessOptimizedQuery = Post::where('status', 'published')
->whereDate('created_at', '>', now()->subDays(30))
->orderBy('created_at', 'desc')
->limit(20)
->get(['id', 'title']); // This still fetches all columns internally
```
0
comments
Follow creator